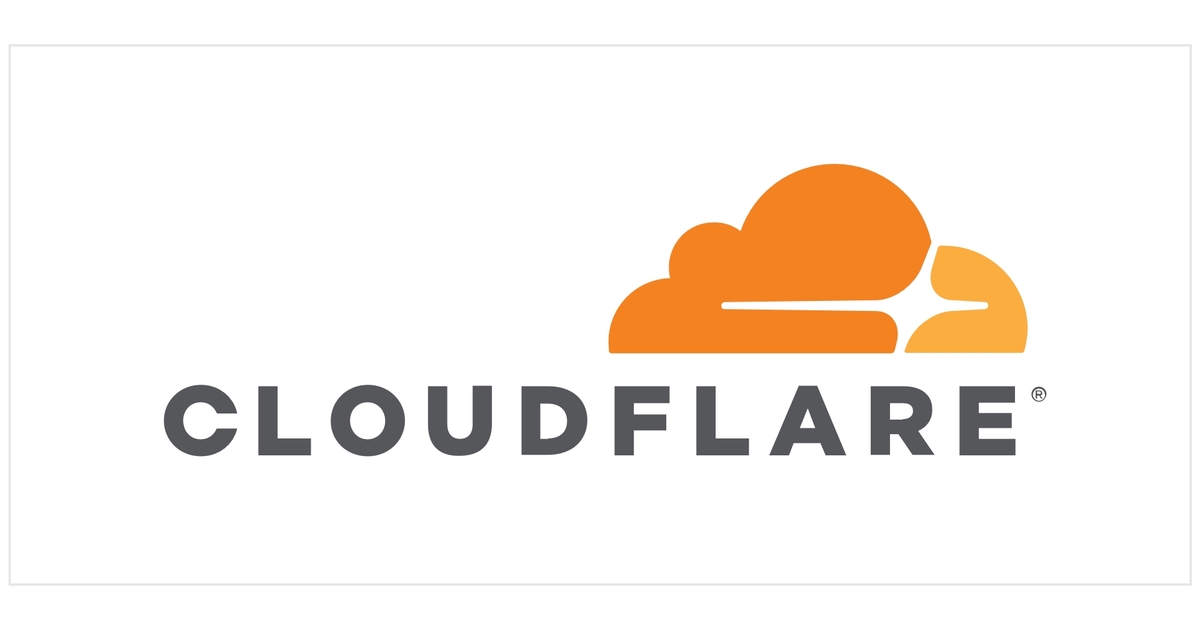
Twitter API with CloudFlare Worker
0x01
For some reason, I can't access Twitter's API directly, therefore, I need a relay.
There are a bunch of "Wheels" for twitter API that you can use in Node.js, BUT sadly, they won't work with Worker.
0x02
This section will shows the key parts of how to tweet by using Twitter API 2.0 from CloudFlare Worker.
wrangler.toml
Add this line to enable nodejs compatibility
1 | node_compat = true |
Import
1 | import OAuth from 'oauth-1.0a'; |
Authentication
It's recommended to use wrangler to manage your api keys
1 | oauth = OAuth({ |
Call API
1 | const endPoint = "https://api.twitter.com/2/tweets" |
本文是原创文章,采用CC BY-NC-SA 4.0协议,完整转载请注明来自Ooooo